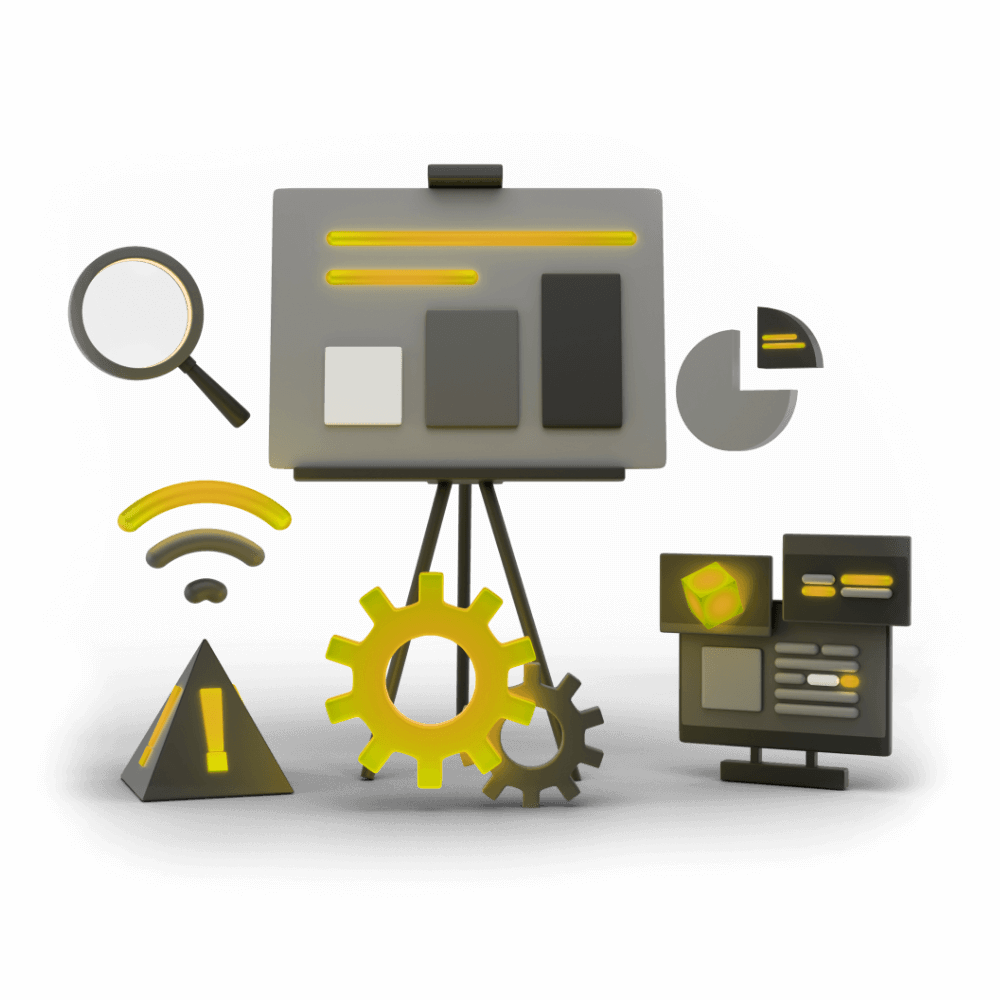
Creating High Performance Ionic Applications
This module focuses of various aspects of creating high performance applications with Ionic and Angular. Topics include how the browser rendering process works, using debugging tools to profile and fix performance, reducing bundle sizes, and architecting code to increase performance.
Faster Change Detection with the OnPush Strategy
Using OnPush to reduce unnecessary change detection cycles
PROModule Outline
- Source Code & Resources PRO
- Lesson 1: Introduction PUBLIC
- Lesson 2: Understanding Browser Rendering PUBLIC
- Lesson 3: Creating Production Builds PRO
- Lesson 4: Measuring Network Requests PRO
- Lesson 5: Debugging Network Issues PRO
- Lesson 6: Measuring Memory PRO
- Lesson 7: Debugging Memory Issues PRO
- Lesson 8: Measuring Frame Rate PRO
- Lesson 9: Debugging Frame Rate Issues PRO
- Lesson 10: Bundle Size & Lazy Loading PRO
- Lesson 11: Interacting with the DOM Efficiently PRO
- Lesson 12: Perceived Performance PRO
- Lesson 13: Dealing with Large Lists PRO
- Lesson 14: Animation Performance PRO
- Lesson 15: Speeding up Observables & Promises PRO
- Lesson 16: Faster Change Detection with the OnPush Strategy PRO
- Lesson 17: Using Web Workers for Heavy Lifting PRO
- Lesson 18: Conclusion PRO
Lesson Outline
Using Immutable Data
If you are not already using immutable data concepts, you might have seen people updating their arrays like this:
public myArray: number[] = [1,2,3];
ngOnInit(){
this.myArray = [...this.myArray, 4];
}
instead of like this:
public myArray: number[] = [1,2,3];
ngOnInit(){
this.myArray.push(4);
}
The first example creates a new array by using the values of the old array with an additional 4
element on the end. The second example just pushes 4
into the existing array. Apart from being a chance to show off cool JavaScript operators like the spread operator, there are practical benefits to using the first approach.
The word immutable just means "not changeable" or "can not be mutated". If you have an "immutable" object you can not change the values of that object. If you want new values, you need to create an entirely new object in memory. We might enforce immutability ourselves by just following some rules, or we might use libraries like immer or Immutable.js to help enforce immutability in our application. This is similar to the philosophy behind why we might use something like TypeScript to help enforce certain rules in our application.
What's the point?
It might just seem like more work to create new objects instead of modifying existing ones. Indeed it is, especially when you are dealing with nested objects it can be quite difficult to update a value in an immutable way.
There are benefits to using immutable data in general, but we are going to focus on one specific example of where using immutable data can help us improve the performance of our Ionic/Angular applications.
In short: we can supply an Angular component with the OnPush
change detection strategy with immutable data to greatly reduce the amount of work Angular needs to do during its change detection cycles.
Change Detection in Angular (in Brief)
In Angular, we bind data values in our templates. When that data is updated, we want the template to reflect those changes. The basic idea with change detection is that we handle updating the data, and Angular will handle reflecting that in our templates for us.
If Angular want to update a template, it needs to know that something has changed. To keep track of this, Angular will know when any of the following events have been triggered within its zone:
- Any browser event (like
click
) - A
setTimeout
callback - A
setInterval
callback - An HTTP request
There are all things that might result in some data changing. Angular will detect when any of these occur and then check the entire component tree for changes. That means that Angular will start at the root component, and make its way through every component in the application to check if the data any of those components rely on has changed.
This probably sounds worse than it is. Change detection in Angular is fast... but it's not free. The larger your application grows the more work there will be for Angular to do during change detection cycles. We can reduce the amount of work Angular has to do but there are some important concepts we need to keep in mind when doing this.
Thanks for checking out the preview of this lesson!
You do not have the appropriate membership to view the full lesson. If you would like full access to this module you can view membership options (or log in if you are already have an appropriate membership).