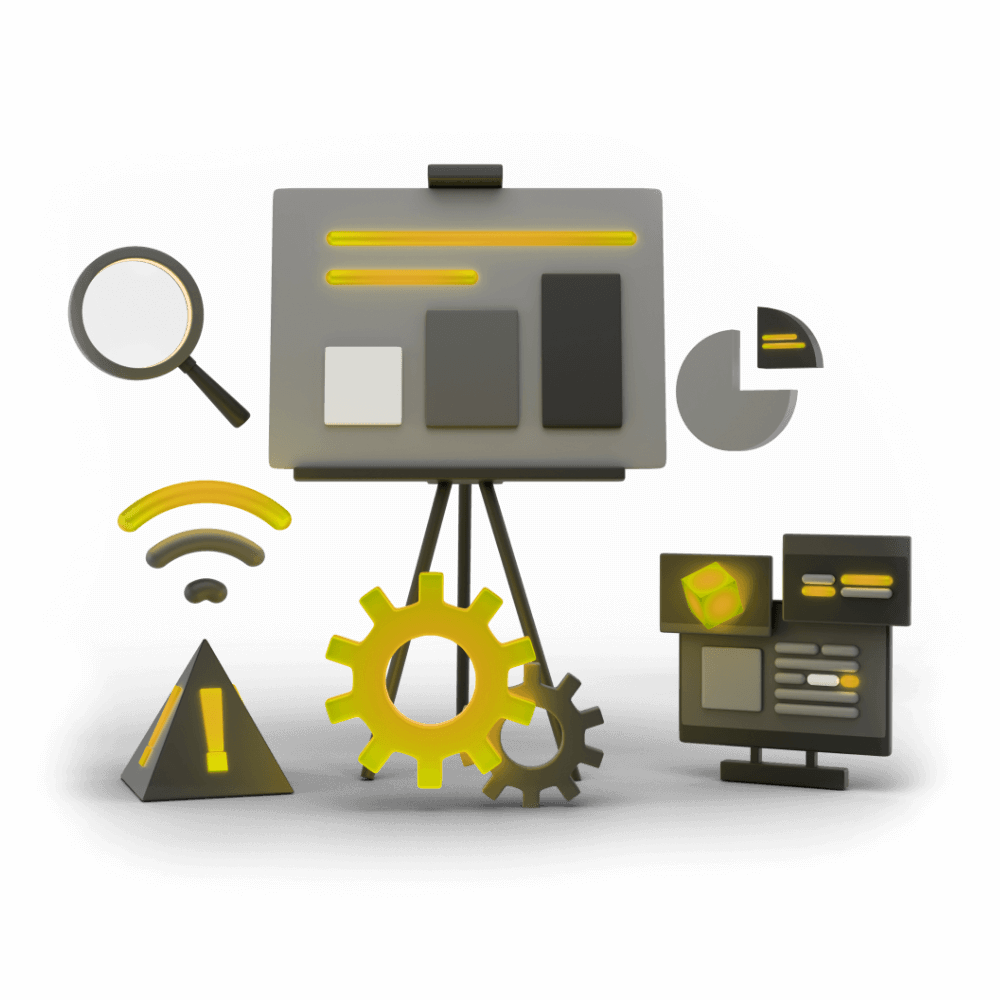
Creating High Performance Ionic Applications
This module focuses of various aspects of creating high performance applications with Ionic and Angular. Topics include how the browser rendering process works, using debugging tools to profile and fix performance, reducing bundle sizes, and architecting code to increase performance.
Speeding up Observables & Promises
Executing promises and observables efficiently
PROModule Outline
- Source Code & Resources PRO
- Lesson 1: Introduction PUBLIC
- Lesson 2: Understanding Browser Rendering PUBLIC
- Lesson 3: Creating Production Builds PRO
- Lesson 4: Measuring Network Requests PRO
- Lesson 5: Debugging Network Issues PRO
- Lesson 6: Measuring Memory PRO
- Lesson 7: Debugging Memory Issues PRO
- Lesson 8: Measuring Frame Rate PRO
- Lesson 9: Debugging Frame Rate Issues PRO
- Lesson 10: Bundle Size & Lazy Loading PRO
- Lesson 11: Interacting with the DOM Efficiently PRO
- Lesson 12: Perceived Performance PRO
- Lesson 13: Dealing with Large Lists PRO
- Lesson 14: Animation Performance PRO
- Lesson 15: Speeding up Observables & Promises PRO
- Lesson 16: Faster Change Detection with the OnPush Strategy PRO
- Lesson 17: Using Web Workers for Heavy Lifting PRO
- Lesson 18: Conclusion PRO
Lesson Outline
Speeding up Observables & Promises
Often in our applications we need to wait on some kind of asynchronous task to complete, and we generally handle the responses from these asynchronous tasks using either Promises or Observables. The great thing about asynchronous tasks is that it allows our application to continue functioning whilst the tasks are completed in the background, and we can even run multiple asynchronous tasks at the same time.
However, it is easy to negatively impact the performance of your application by misusing promises or observables. For the purpose of this lesson, I have created a demo application that contains a service with three methods:
getThingOne(){
return new Promise((resolve) => {
setTimeout(() => {
resolve("thing one");
}, 2000);
});
}
getThingTwo(){
return new Promise((resolve) => {
setTimeout(() => {
resolve("thing two");
}, 2000);
});
}
getThingThree(){
return new Promise((resolve) => {
setTimeout(() => {
resolve("thing three");
}, 2000);
});
}
The purpose of this is to simulate an asynchronous task that takes some time to complete (like a call to a server), in this case each task takes two seconds to complete.
Executing Multiple Promises or Observables
If we wanted to execute multiple promises at the same time we might just do something like this:
ngOnInit(){
this.dataService.getThingOne().then((res) => {
/* do something */
});
this.dataService.getThingTwo().then((res) => {
/* do something */
});
this.dataService.getThingThree().then((res) => {
/* do something */
});
}
No problems here, all of these tasks will execute in parallel and finish at roughly the same time. Meaning after two seconds we will have all of the results we need.
However, it is common for an application to need to wait for some data before continuing with a certain task. To make sure that that task is not executed before the data has been returned from the promise, we generally make a call to that function inside of the .then()
handler, or we could use the async/await
syntax.
Thanks for checking out the preview of this lesson!
You do not have the appropriate membership to view the full lesson. If you would like full access to this module you can view membership options (or log in if you are already have an appropriate membership).