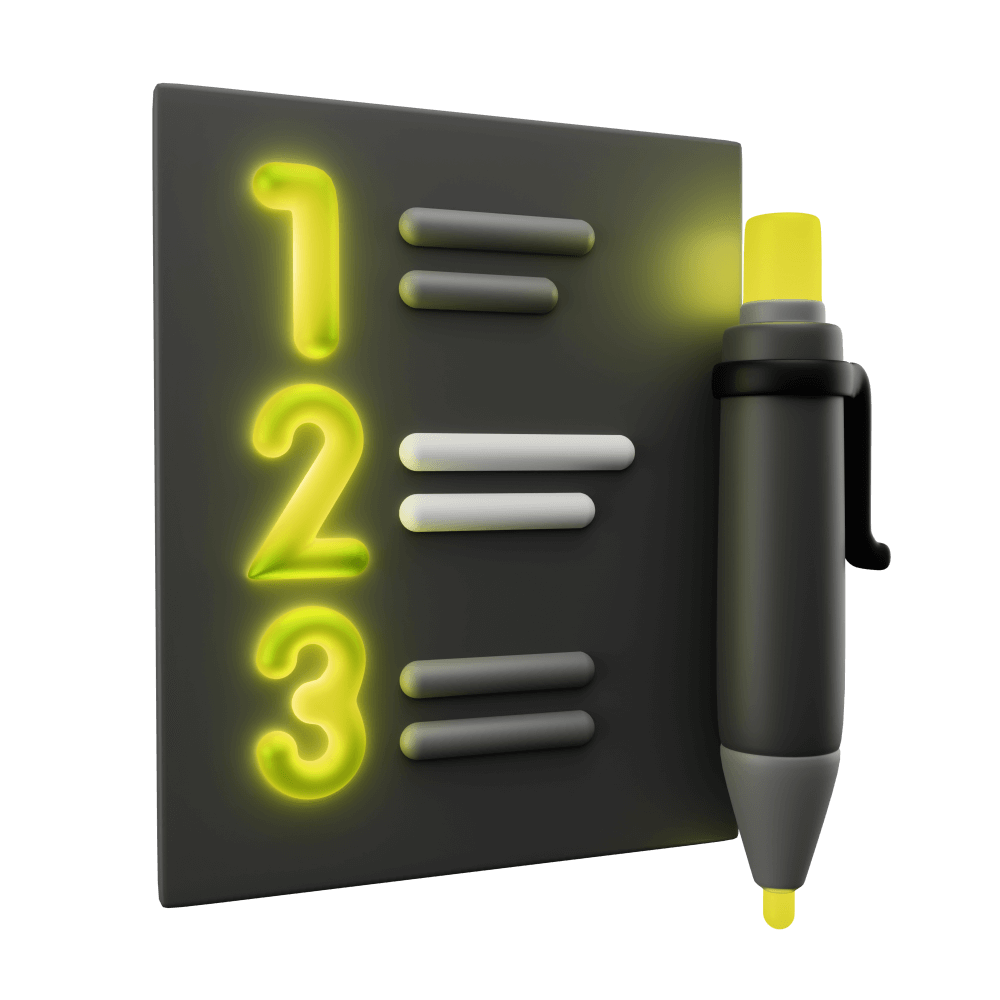
Test Driven Development with Protractor/Jasmine (Legacy)
This module is deprecated and no longer receives updates. Protractor is likely being removed as the default from Angular applications and Protractor itself will likely stop receiving updates and development in the future. I would recommend checking out the Test Driven Development with Cypress/Jest as a replacement.
Testing Asynchronous Code
WARNING: This module is deprecated and no longer receives updates. Protractor is likely being removed as the default from Angular applications and Protractor itself will likely stop receiving updates and development in the future. I would recommend checking out the Test Driven Development with Cypress/Jest as a replacement.
Using fakeAsync to control time and test asynchronous code
DEPRECATEDModule Outline
- Resources PRO
- Lesson 1: Introduction PRO
- Lesson 2: Introduction to Test Driven Development PRO
- Lesson 3: Testing Concepts PRO
- Lesson 4: Jasmine, Karma, and Protractor PRO
- Lesson 5: A Simple Unit Test PRO
- Lesson 6: A Simple E2E Test PRO
- Lesson 7: Introduction to Angular's TestBed PRO
- Lesson 8: Setting up Tests PRO
- Lesson 9: Test Development Cycle PRO
- Lesson 10: Getting Ready PRO
- Lesson 11: The First Tests PRO
- Lesson 12: Injected Dependencies & Spying on Function Calls PRO
- Lesson 13: Building out Core Functionality PRO
- Lesson 14: Testing Asynchronous Code PRO
- Lesson 15: Creating a Mock Backend PRO
- Lesson 16: Setting up the Server PRO
- Lesson 17: Testing Integration with a Server PRO
- Lesson 18: Testing Storage and Reauthentication PRO
- Lesson 19: Refactoring with Confidence PRO
- Lesson 20: Conclusion PRO
Lesson Outline
Testing Asynchronous Code
We're getting into some more complicated situations now, so before we continue with developing the application we are going to spend the next couple of lessons covering a couple more testing concepts.
Up until this point our tests have been reasonably simple, we have just been dealing with calling functions and passing data between pages. All the code we have been testing has been synchronous, which means everything has been getting executed one line after the other and we can just trigger whatever function we are interested in, and then test that something happened.
It gets a little more complicated when we start testing asynchronous code. Unlike synchronous code that gets executed right away, asynchronous code will get executed at some time after all the rest of our synchronous code has run. If we wanted to write a test that relied on the result of an asynchronous function like the following test:
it('someThing should be true after someAsynchronousFunction runs', () => {
component.someAsynchronousFunction();
expect(component.someThing).toBe(true);
});
Assuming that someAsynchronousFunction
sets someThing
to true after some asynchronous operation like resolving a promise, we are going to have issues when running this test.
The flow of our test would look something like this:
- Call
someAsynchronousFunction
- Check that
someThing
istrue
- Waiting for promise to resolve...
someThing
is set totrue
Clearly, this is not what we want. We need someThing
to be set to true
before we check it with our expect
statement. What we really want is this:
- Call
someAsynchronousFunction
- Waiting for promise to resolve...
someThing
is set totrue
- Check that
someThing
istrue
In order to deal with this situation, we can run the test inside of fakeAsync
which essentially gives us the ability to control time in our tests.
fakeAsync, flushMicrotasks, and tick
Thanks for checking out the preview of this lesson!
You do not have the appropriate membership to view the full lesson. If you would like full access to this module you can view membership options (or log in if you are already have an appropriate membership).