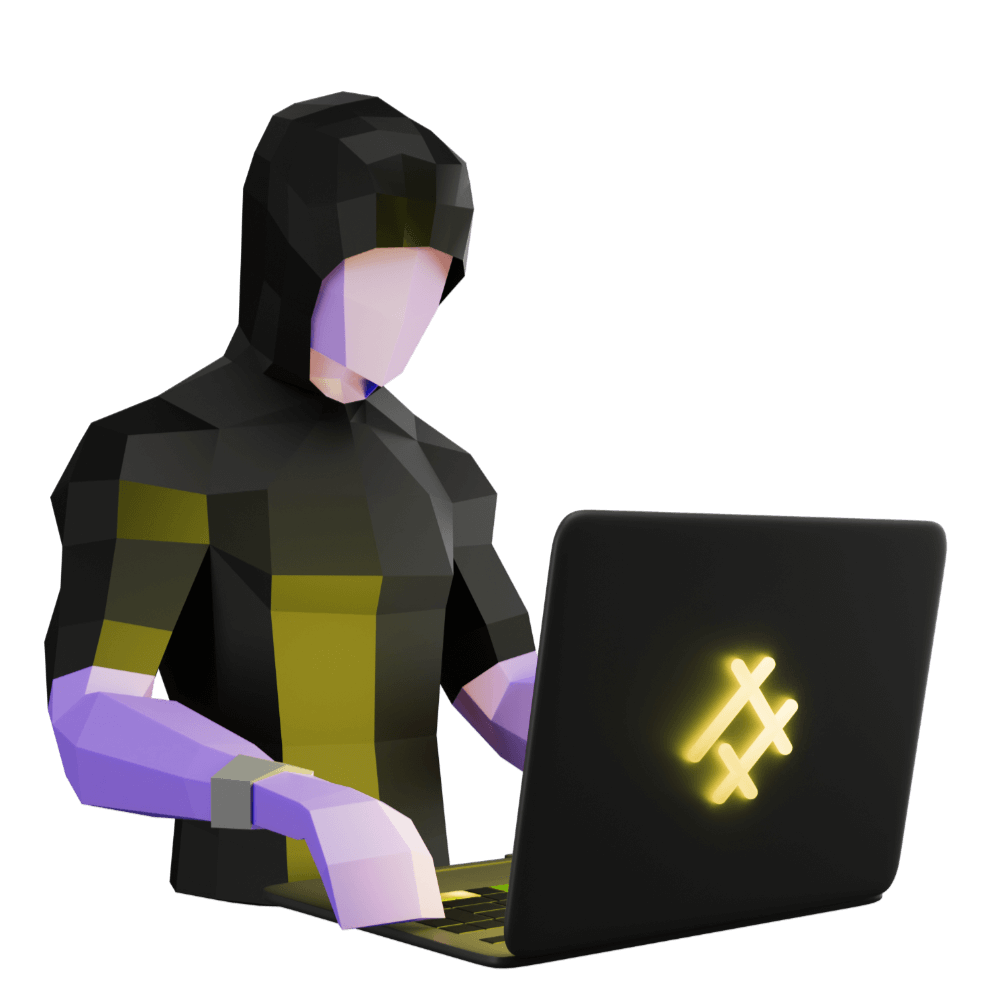
Creating a Modern Firebase Powered Application with TDD
Use reactive programming and tests to build a professional app
[Sprint Four] - View Survey
Adding the ability for the admin to view surveys
PROModule Outline
- Source Code & Resources PRO
- Lesson 1: Introduction PUBLIC
- Lesson 2: The Structure of this Module PUBLIC
- Lesson 3: [Sprint One] Setting up Firebase PUBLIC
- Lesson 4: [Sprint One] Creating Security Rules with TDD PRO
- Lesson 5: [Sprint One] Testing Authentication PRO
- Lesson 6: [Sprint One] Component Store PRO
- Lesson 7: [Sprint One] Circumventing Firebase Authentication for E2E Tests PRO
- Lesson 8: [Sprint Two] Displaying Client List from Firestore PRO
- Lesson 9: [Sprint Two] - Adding Clients PRO
- Lesson 10: [Sprint Two] - Editing Clients PRO
- Lesson 11: [Sprint Two] - Client Details PRO
- Lesson 12: Preparing for Delivery PRO
- Lesson 13: Configuring for Production PRO
- Lesson 14: [Sprint Three] - Refactoring PRO
- Lesson 15: [Sprint Three] Setting up PWA PRO
- Lesson 16: [Sprint Three] Logout PRO
- Lesson 17: [Sprint Three] Delete a Client PRO
- Lesson 18: [Sprint Three] - Feedback Mechanism PRO
- Lesson 19: [Sprint Three] View Feedback PRO
- Lesson 20: More Styling PRO
- Lesson 21: [Sprint Four] - Refactoring Feedback PRO
- Lesson 22: [Sprint Four] - Feedback Dates PRO
- Lesson 23: [Sprint Four] - Client Survey PRO
- Lesson 24: [Sprint Four] - View Survey PRO
- Lesson 25: Final Touches PRO
- Lesson 26: Conclusion PRO
Lesson Outline
Feat: Results of the users questionnaire to be visible
Now we just need to finish off this feature by adding the ability for the admin to view the results. This will be the last user story that we work on for this module!
User story: As a massage therapist, I want the results of the users questionnaire to be visible to me, so that I have the information required for the massage and so that I can get them to verify the information in person
Project management
Remember to move the card for this task to the Test
column, and create a new task branch to work on.
We have our survey/history forms being stored in the client document, but now we need a way for the massage therapist to actually view those. This is going to be a little different than the ability to view the feedback form because there could potentially be multiple survey submissions associated with each client. The basic idea of what we would want is for the admin to be able to view a particular client, then from within that client select to view the survey responses, then show a list of all of the responses that have been submitted for that client, and then provide the ability to view the responses for one specific submission.
This would likely need to be extended in the future as well such that the massage therapist could edit these responses or have the client fill them out through the admin interface on site, but for now we are just going to focus on the data display aspect of it.
Once again, we will start with our E2E test in the client-detail.cy.ts
file:
it('can view responses to the client history form for individual clients', () => {
cy.callFirestore('set', 'clients/abc123', testClient);
getViewSurveyResponsesButton().click();
getSurveyResponseList().first().click();
getRenderJsonValue().should('have.value', 'someValue');
});
We will also need to update the test client in this file to include the survey
field:
const testClient = {
name: {
first: 'Josh',
last: 'Morony',
},
phone: '333',
email: '[email protected]',
notes: '',
survey: ['{"someProperty": "someValue"}'],
};
and we will also need to define the following new utility methods:
export const getViewSurveyResponsesButton = () =>
cy.get('[data-test="view-survey-responses-button"]');
export const getSurveyResponseList = () => cy.get('[data-test="survey-list"]');
export const getViewSurveyResponsesBackButton = () =>
cy.get('[data-test="view-survey-responses-back-button"]');
export const getViewSurveyResponseDetailBackButton = () =>
cy.get('[data-test="view-survey-response-detail-back-button"]');
Now let's check that it fails:
Timed out retrying after 4000ms: Expected to find element: [data-test="view-survey-responses-button"], but never found it.
It can't find the survey button so it fails. We are going to add that, but we are also going to add the two additional pages we require for this feature: the client-survey
page and the client-survey-detail
page:
ionic g page clients/feature/ClientSurvey --module clients/feature/client-detail/client-detail-routing.module --route-path="history"
ionic g page clients/feature/ClientSurveyDetail --module clients/feature/client-survey/client-survey-routing.module --route-path=":index"
For the client-survey
page we want to be able to view the responses for a specific client, and for the client-survey-detail
page we want to be able to retrieve a specific index from the survey
array for a specific client - so we need both the :id
(for the client) and :index
(for the survey response array) values which we can get through the route. You might notice that the route we have created for our client-survey
page:
const routes: Routes = [
{
path: '',
component: ClientSurveyPage
},
{
path: ':index',
loadChildren: () => import('../client-survey-detail/client-survey-detail.module').then( m => m.ClientSurveyDetailPageModule)
}
];
Only includes the :index
param, but we will be able to get the :id
from the parent route. The full path to a survey response will look like this:
clients/123/history/1
Now we can add that button into the client-detail
page:
Thanks for checking out the preview of this lesson!
You do not have the appropriate membership to view the full lesson. If you would like full access to this module you can view membership options (or log in if you are already have an appropriate membership).