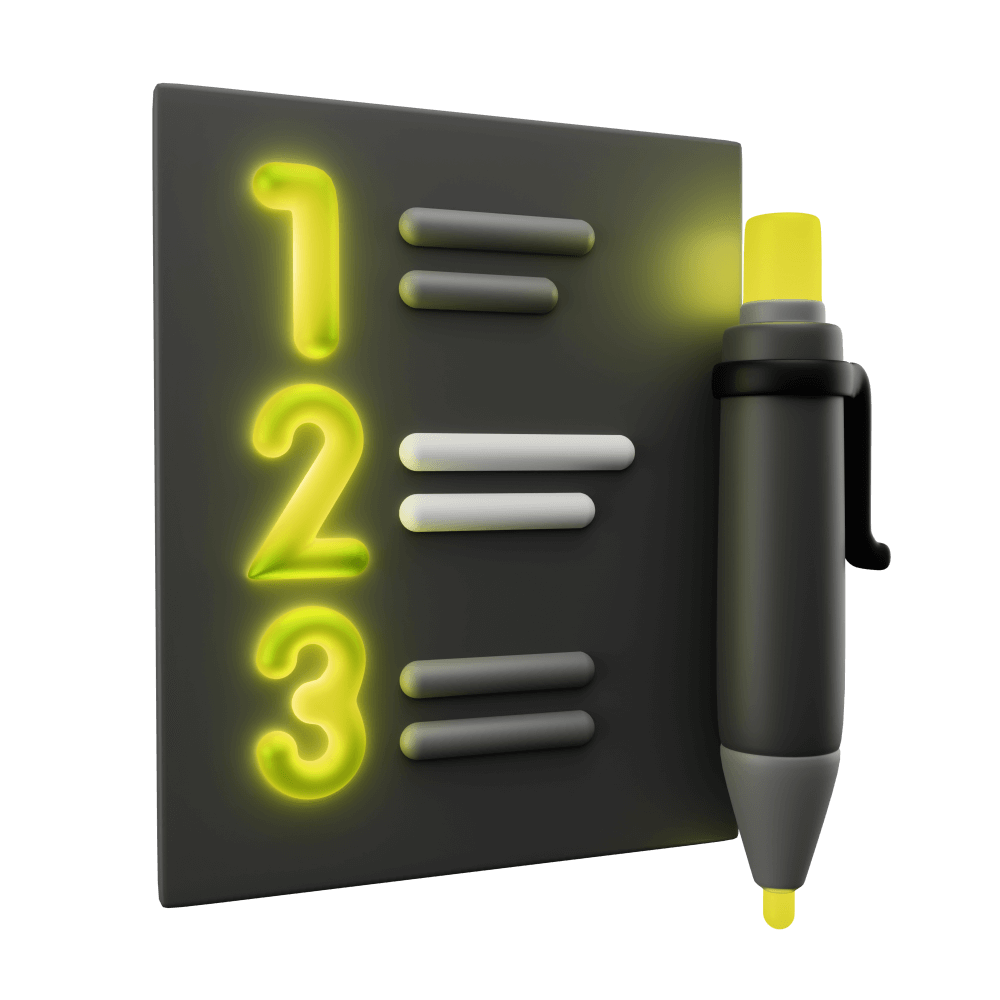
Test Driven Development with Protractor/Jasmine (Legacy)
This module is deprecated and no longer receives updates. Protractor is likely being removed as the default from Angular applications and Protractor itself will likely stop receiving updates and development in the future. I would recommend checking out the Test Driven Development with Cypress/Jest as a replacement.
Introduction to Test Driven Development
WARNING: This module is deprecated and no longer receives updates. Protractor is likely being removed as the default from Angular applications and Protractor itself will likely stop receiving updates and development in the future. I would recommend checking out the Test Driven Development with Cypress/Jest as a replacement.
Introducing automated tests and the concept of TDD
DEPRECATEDModule Outline
- Resources PRO
- Lesson 1: Introduction PRO
- Lesson 2: Introduction to Test Driven Development PRO
- Lesson 3: Testing Concepts PRO
- Lesson 4: Jasmine, Karma, and Protractor PRO
- Lesson 5: A Simple Unit Test PRO
- Lesson 6: A Simple E2E Test PRO
- Lesson 7: Introduction to Angular's TestBed PRO
- Lesson 8: Setting up Tests PRO
- Lesson 9: Test Development Cycle PRO
- Lesson 10: Getting Ready PRO
- Lesson 11: The First Tests PRO
- Lesson 12: Injected Dependencies & Spying on Function Calls PRO
- Lesson 13: Building out Core Functionality PRO
- Lesson 14: Testing Asynchronous Code PRO
- Lesson 15: Creating a Mock Backend PRO
- Lesson 16: Setting up the Server PRO
- Lesson 17: Testing Integration with a Server PRO
- Lesson 18: Testing Storage and Reauthentication PRO
- Lesson 19: Refactoring with Confidence PRO
- Lesson 20: Conclusion PRO
Lesson Outline
DEPRECATION WARNING
IMPORTANT: This module is deprecated and is only kept for legacy reasons. It will not receive any more updates. As of May 2021 Protractor will no longer be included by default in new Angular projects, and the development of Protractor will eventually cease completely. Instead, you can use Cypress to test your Ionic/Angular projects.
The only reason that you should go through this module is if you need to maintain a legacy Angular application that is still using Protractor. If you want to learn how to use Test Driven Development with Ionic and Angular you should instead start the Cypress/Jest module also available in Elite Ionic.
Introduction to Test Driven Development
Test Driven Development is all about using automated tests to drive the development of your application, rather than writing your application's code first and writing tests after. In its simplest form, the process for Test Driven Development looks like this:
- Write a test for functionality that you want to build
- Check that the test fails
- Implement code to satisfy the test
- Check that the test passes
- Repeat
Before we get into the specifics of Test Driven Development, let's take a few steps back and discuss what exactly automated tests are.
What are Automated Tests?
An automated test, put simply, is code that tests your code. We generally use a testing framework to help us write these tests, but you will write blocks of code that trigger the code you want to test, and then check the result.
Let's see what that would look like with just plain Javascript (i.e. without a framework that helps build/run tests):
// Test that `incrementTotal()` increases the total by 1
let myTestObject = new SomeObject();
let oldTotal = myTestObject.getTotal();
myTestObject.incrementTotal();
let newTotal = myTestObject.getTotal();
if (newTotal === oldTotal + 1) {
console.log('test passed!');
} else {
console.log('test failed!');
}
The above code will test if the incrementTotal()
function increases the total
on SomeObject
by 1
. If the code is working as it should we would see "test passed!" logged to the console, but if it isn't we will see "test failed!". This test doesn't add any functionality to our application, it is purely for checking if parts of our application are doing what they are supposed to be doing.
Thanks for checking out the preview of this lesson!
You do not have the appropriate membership to view the full lesson. If you would like full access to this module you can view membership options (or log in if you are already have an appropriate membership).