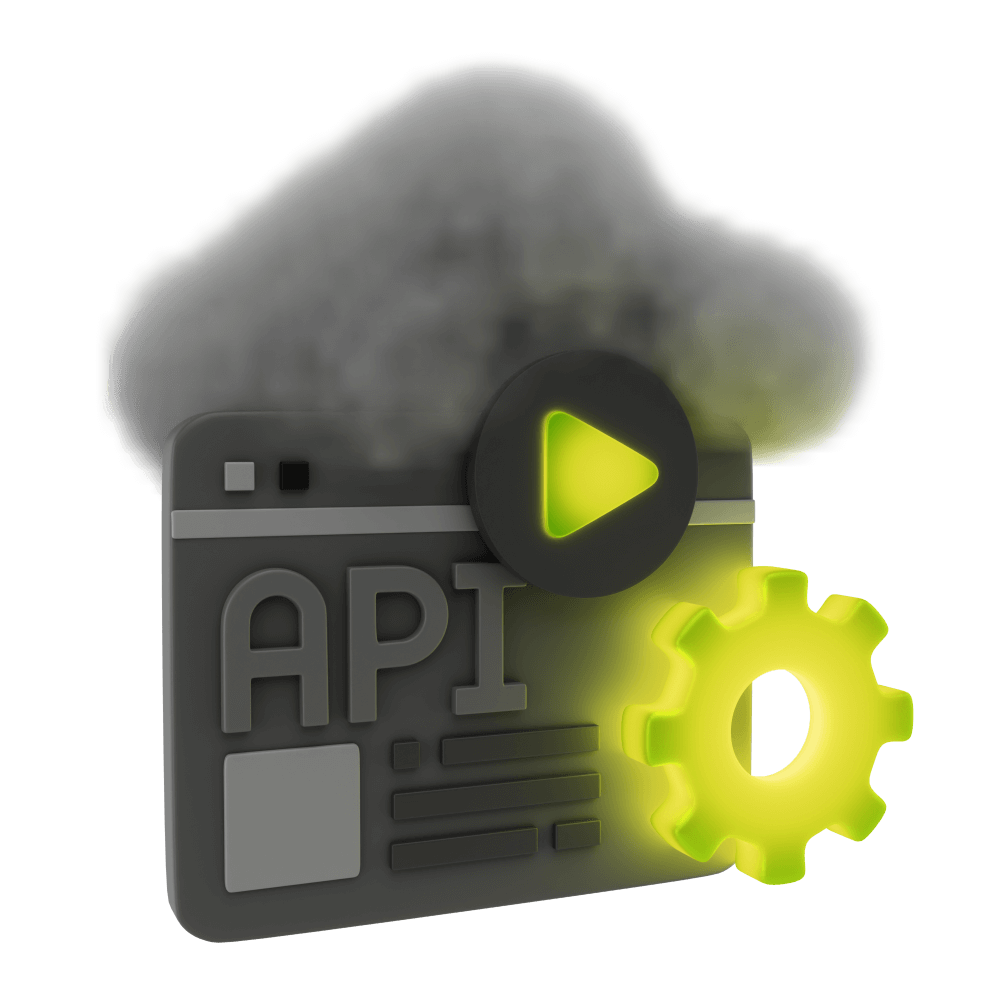
Offline/Online Sync in a Real World Ionic Application with CouchDB
This is supposed to be a longer description
Advanced Form Validation
Creating advanced validations for the register form
PROModule Outline
- Source Code & Resources PRO
- Lesson 1: Introduction PUBLIC
- Lesson 2: Application Requirements PUBLIC
- Lesson 3: A Brief Introduction to NoSQL PUBLIC
- Lesson 4: Introduction to CouchDB PRO
- Lesson 5: Introduction to PouchDB PRO
- Lesson 6: Structuring Data in CouchDB PRO
- Lesson 7: Installing CouchDB Locally PRO
- Lesson 8: Adding Data to Futon PRO
- Lesson 9: Starting the Application PRO
- Lesson 10: Setting up the Basic User Interface PRO
- Lesson 11: Using Design Documents to Create Views in CouchDB PRO
- Lesson 12: Getting Data From CouchDB into Ionic PRO
- Lesson 13: Using Node, Express, and SuperLogin PRO
- Lesson 14: Login and Registration PRO
- Lesson 15: Offline Access and Reauthentication PRO
- Lesson 16: Advanced Form Validation PRO
- Lesson 17: Restricting Document Updates PRO
- Lesson 18: Filtering Data from CouchDB PRO
- Lesson 19: Improving User Experience PRO
- Lesson 20: Migrating to Production PRO
- Lesson 21: Conclusion PRO
Lesson Outline
Advanced Form Validation
The form we added to the register page uses Angular's FormBuilder
to create a group of fields, and we've already set up some basic validations on those fields.
For the purpose of this lesson, I will assume that you are already familiar with creating forms with FormBuilder
, but if you are not I would recommend taking a look at this blog post. The important thing to understand is that a FormGroup
consists of FormControls
.
A FormControl
is tied to an input field, it has a value (i.e. the value the user has entered), and a validation state (i.e. whether or not the value is valid based on an optional validation function). Since each form control has a validation state, we can easily detect whether or not the form is valid, which allows us to do things like this:
if(this.registerForm.valid)
or this:
*ngIf="!registerForm.valid"
We can easily modify the interface or the behaviour of our application based on whether or not fields in the form are valid. In this lesson, we are going to cover the different kinds of validators we can use which will determine whether or not our form fields are valid.
There are primarily two types of validators: synchronous validators and asynchronous validators. Let's talk through the differences between those.
Synchronous Validators
A synchronous validator uses synchronous code to verify whether or not a field is valid. This means that the validation happens immediately, and it is generally the simpler of the two types of validators. We already have some synchronous validators defined in our register page, so let's take a look at those:
username: ['', Validators.compose([Validators.maxLength(16), Validators.pattern('[a-zA-Z0-9]*'), Validators.required])],
email: ['', Validators.compose([Validators.maxLength(30), Validators.required])],
password: ['', Validators.compose([Validators.maxLength(30), Validators.required])],
confirmPassword: ['', Validators.compose([Validators.maxLength(30), Validators.required])]
Synchronous validators are supplied as the second parameter (the fields value is the first parameter) - we don't have a third parameter just yet but we will in a moment when we add asynchronous validators. Let's take a look specifically at the validators for the username
FormControl:
username: ['', Validators.compose([Validators.maxLength(16), Validators.pattern('[a-zA-Z0-9]*'), Validators.required])],
We are just using the default Validators
that Angular provides, so we are using:
Validators.maxLength(16)
to enforce that the maximum length of this field is 16 charactersValidators.pattern('[a-zA-Z0-9]*')
to enforce a regex pattern on this field - this will only allow letters and numbersValidators.required
to enforce that this field is required
Since we have multiple validators we use Validators.compose
to supply an array of all of the validators that we want to use for this form control. You can find other validators in the documentation, or you can also create your own custom validators (which we will be doing for our asynchronous validators in a moment).
If any of the conditions for these validators are not met, then the form control will be marked as invalid.
Asynchronous Validators
Asynchronous validators are basically identical in concept to synchronous validators, except for the fact that the validation runs asynchronously. This means that our application will continue executing other code whilst our validation function runs some asynchronous code, like a request to a server.
This is exactly what we are going to do now, we are going to write some custom asynchronous validators that will make a request to our server to verify usernames and emails (ensuring that they are not already in use).
Create an Email Validator
Thanks for checking out the preview of this lesson!
You do not have the appropriate membership to view the full lesson. If you would like full access to this module you can view membership options (or log in if you are already have an appropriate membership).