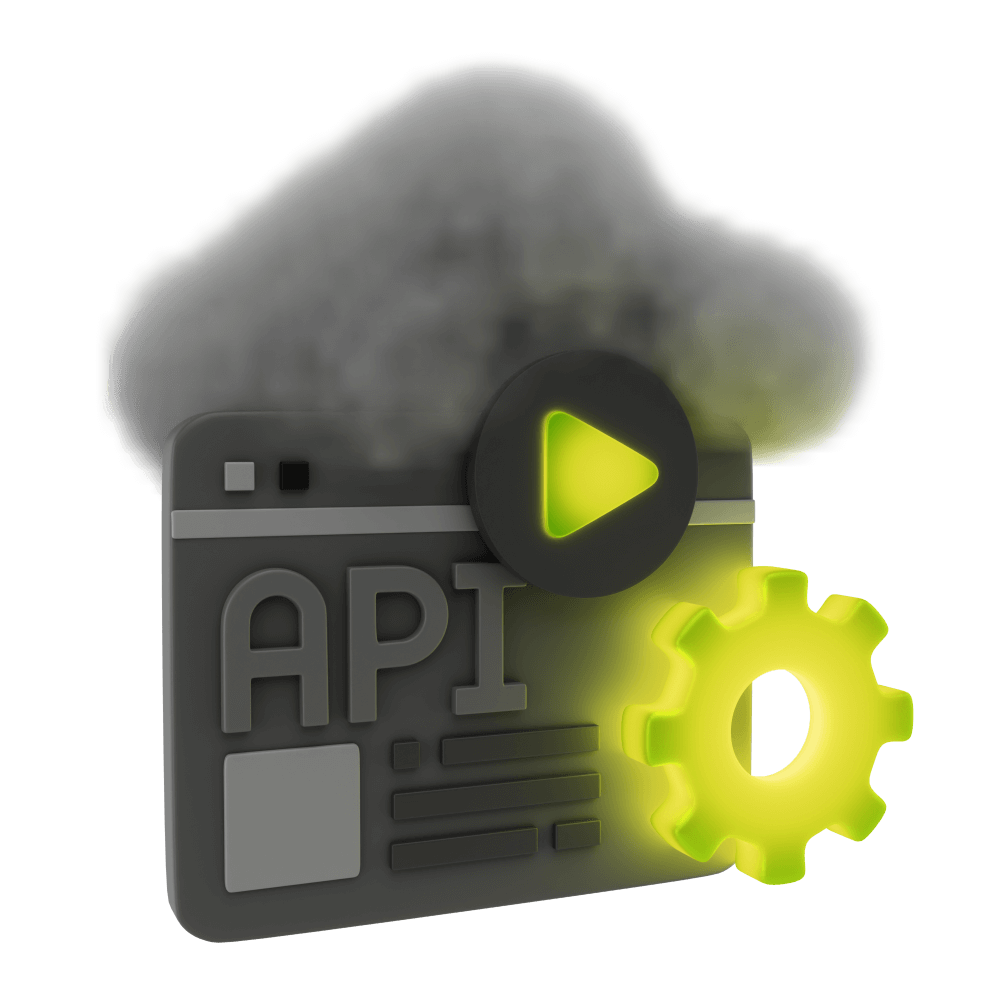
Offline/Online Sync in a Real World Ionic Application with CouchDB
This is supposed to be a longer description
Introduction to CouchDB
An introduction to the basics of CouchDB
PROModule Outline
- Source Code & Resources PRO
- Lesson 1: Introduction PUBLIC
- Lesson 2: Application Requirements PUBLIC
- Lesson 3: A Brief Introduction to NoSQL PUBLIC
- Lesson 4: Introduction to CouchDB PRO
- Lesson 5: Introduction to PouchDB PRO
- Lesson 6: Structuring Data in CouchDB PRO
- Lesson 7: Installing CouchDB Locally PRO
- Lesson 8: Adding Data to Futon PRO
- Lesson 9: Starting the Application PRO
- Lesson 10: Setting up the Basic User Interface PRO
- Lesson 11: Using Design Documents to Create Views in CouchDB PRO
- Lesson 12: Getting Data From CouchDB into Ionic PRO
- Lesson 13: Using Node, Express, and SuperLogin PRO
- Lesson 14: Login and Registration PRO
- Lesson 15: Offline Access and Reauthentication PRO
- Lesson 16: Advanced Form Validation PRO
- Lesson 17: Restricting Document Updates PRO
- Lesson 18: Filtering Data from CouchDB PRO
- Lesson 19: Improving User Experience PRO
- Lesson 20: Migrating to Production PRO
- Lesson 21: Conclusion PRO
Lesson Outline
Introduction to CouchDB
We've already introduced the concept of a NoSQL database - a database that is not relational. There are many different types of NoSQL databases, and we know that CouchDB is a document based NoSQL database. This means that it stores its data as JSON objects called "documents" that look like this:
{
"_id": 1,
"name": 'Josh',
"country": 'Australia',
"interests": ['Ionic', 'Writing', 'Travelling']
}
We're going to be working with CouchDB quite extensively in this module, so we're going to need to get a little more familiar with it than that. As we established, there are a lot of different types of NoSQL databases, but then there are even different types of document based databases as well. You would have likely heard of Firebase, and that is also a document based database, but there are a lot of differences between Firebase and CouchDB even though they are both document based.
In this lesson we are going to get acquainted with some of CouchDB's inner workings.
Syncing and Offline Data
There are many advantages to using CouchDB including the ease of which it can be scaled, and the speed of read and write operations, but the killer feature when it comes to mobile applications is its ability to synchronise between multiple databases.
Synchronising two or more databases can be astoundingly difficult, and in order to provide offline functionality in an application, we need to provide local data to the user that can be read and modified, and then later synced back to the main database or databases when a network connection becomes available.
When using CouchDB, it is trivially easy to do this. CouchDB can run on just about anything, so we could potentially have CouchDB databases all over the place all syncing with each other. If we want to allow a user access to data whilst they are offline and then sync that data when they are online, we could have one CouchDB database running locally on the device, and another CouchDB database hosted on a server somewhere.
Interacting with a CouchDB Database
A CouchDB database implements a RESTful API, which means we can interact with it using HTTP methods like GET
, PUT
, POST
, and DELETE
. If we wanted to read some data from a CouchDB database we might make a GET
request to the following URL:
http://someserver.com/mydatabase/_design/posts/_view/by_date_published
to retrieve some blog posts. We can also use this RESTful API to trigger the replication functionality. All we would have to do is fire off a POST
request with the following data:
{"source":"http://example.org/example-database","target":"http://admin:[email protected]:5984/example-database", "continuous":true}
We're telling CouchDB to replicate the database at the first URL to the database at the second. This is an extremely complex problem made extremely easy to implement - you can literally make one simple POST
request and the job is done. I wanted to show you that you can interact with a CouchDB database directly using the HTTP API, but we will be using PouchDB (more on that later) throughout this module to help us interact with CouchDB.
When using the PouchDB library in your Ionic application, you could trigger a sync between a local database (i.e. one running on the users phone) and a remote database (running on a server) with a single line of code:
PouchDB.sync('mydb', 'http://url/to/remote/database');
This is likely a more comfortable syntax for most people, rather than manually sending off HTTP requests with JSON configurations.
Data Structure
We've already touched on the document based data structure, but now we are going to talk about it in a little more depth.
Data in CouchDB, like in many (but not all) NoSQL databases, is stored as documents and there is no pre-defined schema. Unlike a relational database where we need to describe what the structure of our data is before we insert it (a schema), and can only insert data that matches that schema, with CouchDB you can store whatever kind of data you want, whenever you want.
Thanks for checking out the preview of this lesson!
You do not have the appropriate membership to view the full lesson. If you would like full access to this module you can view membership options (or log in if you are already have an appropriate membership).