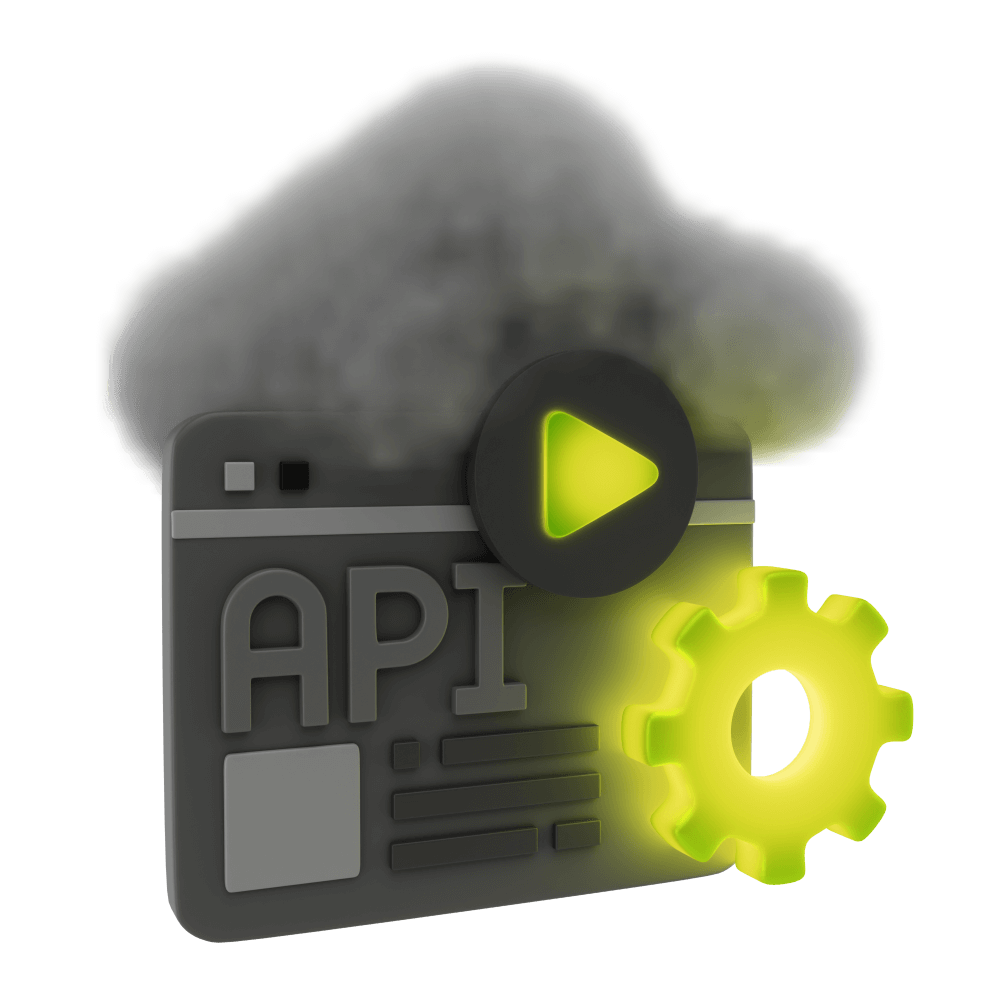
Offline/Online Sync in a Real World Ionic Application with CouchDB
This is supposed to be a longer description
Setting up the Basic User Interface
Creating the basic user interface for the application
PROModule Outline
- Source Code & Resources PRO
- Lesson 1: Introduction PUBLIC
- Lesson 2: Application Requirements PUBLIC
- Lesson 3: A Brief Introduction to NoSQL PUBLIC
- Lesson 4: Introduction to CouchDB PRO
- Lesson 5: Introduction to PouchDB PRO
- Lesson 6: Structuring Data in CouchDB PRO
- Lesson 7: Installing CouchDB Locally PRO
- Lesson 8: Adding Data to Futon PRO
- Lesson 9: Starting the Application PRO
- Lesson 10: Setting up the Basic User Interface PRO
- Lesson 11: Using Design Documents to Create Views in CouchDB PRO
- Lesson 12: Getting Data From CouchDB into Ionic PRO
- Lesson 13: Using Node, Express, and SuperLogin PRO
- Lesson 14: Login and Registration PRO
- Lesson 15: Offline Access and Reauthentication PRO
- Lesson 16: Advanced Form Validation PRO
- Lesson 17: Restricting Document Updates PRO
- Lesson 18: Filtering Data from CouchDB PRO
- Lesson 19: Improving User Experience PRO
- Lesson 20: Migrating to Production PRO
- Lesson 21: Conclusion PRO
Lesson Outline
Setting up the Basic User Interface
Our first goal is going to be to get the basic interface set up for the application, this means we will need to implement the following:
- A notices page that displays a list of notices
- An add notice page that allows for the creation of new notices
- A chat page that displays a list of chats, and allows for the creation of new chats
We will be implementing a simple version to begin with that will not actually allow us to add data, we won't be worrying about integrating with PouchDB and CouchDB just yet. We also won't be worrying too much about the design or user experience just yet, our main focus is to get the bare bones interface in place.
Once we have that we can start getting into the specifics of CouchDB and PouchDB and how we can go about integrating that nicely into the architecture of our Ionic application. Then when we have the basics working how we want, we can circle back to improving the interface.
Set up Tabs
Since we didn't use the tabs
starter we will need to do just a little bit of configuration to get our tabs up and running.
Modify src/app/home/home-routing.module.ts to reflect the following:
import { NgModule } from '@angular/core';
import { RouterModule, Routes } from '@angular/router';
import { HomePage } from './home.page';
const routes: Routes = [
{
path: 'tabs',
component: HomePage,
children: [
{
path: 'notices',
children: [
{
path: '',
loadChildren: () =>
import('../notices/notices.module').then(
(m) => m.NoticesPageModule
),
},
],
},
{
path: 'chat',
children: [
{
path: '',
loadChildren: () =>
import('../chat/chat.module').then((m) => m.ChatPageModule),
},
],
},
],
},
{
path: '',
redirectTo: '/tabs/notices',
pathMatch: 'full',
},
];
@NgModule({
imports: [RouterModule.forChild(routes)],
exports: [RouterModule],
})
export class HomePageRoutingModule {}
We just have our Notices
and Chat
tabs defined here. Now we just need to add the Ionic tabs components to our home pages template.
Modify src/app/home/home.page.html to reflect the following:
<ion-tabs>
<ion-tab-bar color="primary" slot="top">
<ion-tab-button tab="notices">
<ion-icon name="clipboard"></ion-icon>
</ion-tab-button>
<ion-tab-button tab="chat">
<ion-icon name="chatbubbles"></ion-icon>
</ion-tab-button>
</ion-tab-bar>
</ion-tabs>
Notice that we have supplied slot="top"
so that our tab bar will be displayed at the top of the application rather than the bottom. The reason we are doing this is because we will be adding a chat input at the bottom of the chat page, so the tab bar works better at the top in this instance. Users would expect a chat input to be at the bottom of the screen so it is a good idea to conform to this, but it is also easier to interact with elements closer to the bottom of the screen, and the user will likely interact with the chat input more than they do the tab bar.
Displaying Notices
The first tab is our notices page, and we will be implementing a card list on this page that will display all the notices. For now, we are just going to set up some dummy data in the constructor that we will replace with real data from CouchDB later.
So that we can give our notices an appropriate type, let's create an interface for it first.
Create a file at src/app/interfaces/notice.ts and add the following:
export interface Notice {
title: string;
message: string;
author: string;
}
Since we will be launching a modal based on our AddNoticePage
from our notices page, we will need to add the AddNoticePage
as a declaration
to the module for our notices page so that we can supply it to the ModalController
. Let's do that now.
Thanks for checking out the preview of this lesson!
You do not have the appropriate membership to view the full lesson. If you would like full access to this module you can view membership options (or log in if you are already have an appropriate membership).