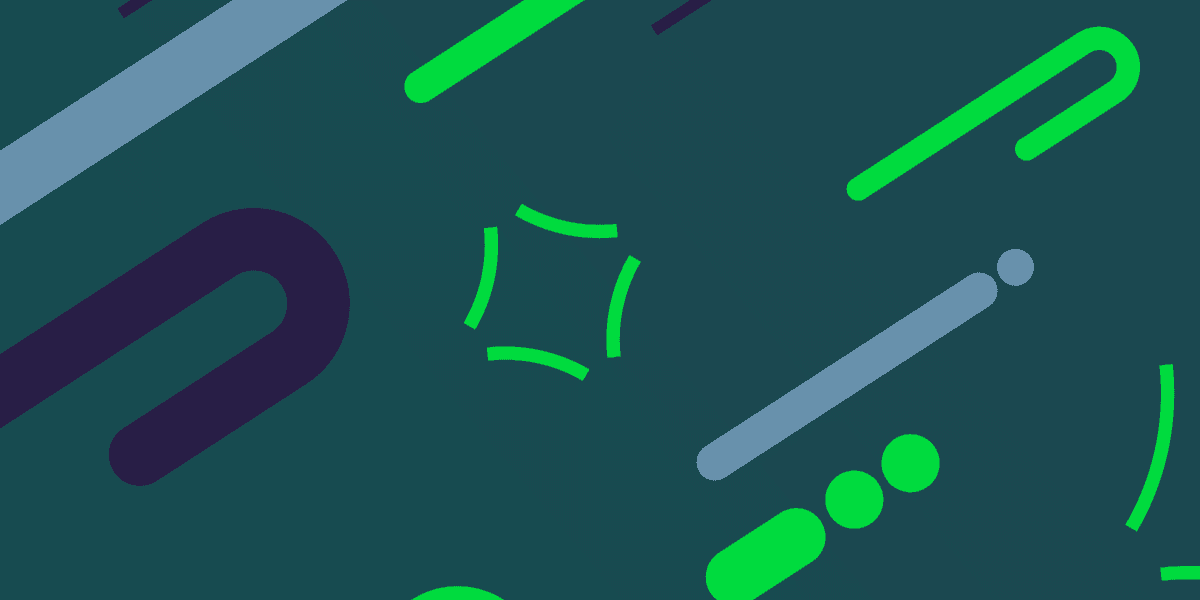
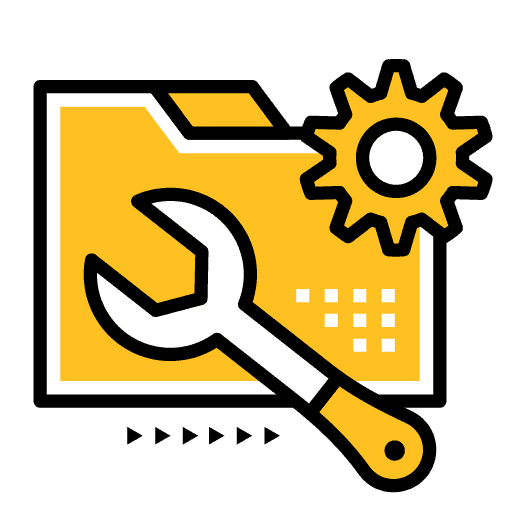
Using HTML File Input for Uploading Native iOS/Android Files
In the last tutorial, we covered how to handle file uploads in Ionic using the <input type="file">
element. This included using the file input element to grab a reference to a file and then upload that file to a Node/Express server (there is also an extension to the tutorial available where we build the backend with NestJS instead).
Those tutorials focused on a desktop/web environment, but what do we do to handle file uploads when our applications are deployed natively to iOS or Android? Can we still use <input type="file">
?
The answer to that question is mostly yes, but there are a few things to keep in mind.
Outline
Before we get started
If you have not already read (or watched) the previous tutorial, it would be a good idea to complete it before reading this one. The previous tutorial provides a lot of important context around how the HTML <input>
elements works when specifying the file
type, and also around how those files can be uploaded to a backend server with multipart/form-data
and the FormData
API.
What is the difference between web and native for file uploads?
When we use the <input type="file">
element in a standard desktop/web environment, we can be quite certain of its behaviour. We click the Choose file
button and a file explorer window is launched where we can select any file on our computer.
When we try to do this on mobile the behaviour is quite different and exactly how it behaves will depend on the platform. Generally speaking, the process is still more or less the same - the user clicks the button, selects a file, and then we are able to get a reference to that file. However, we don't have a standard "file explorer" window that pops up and allows the user to select any file on their device. Depending on the context, the camera might be launched directly, or the user might be prompted to choose a file directly from the file system, or the user might be offered a choice between browsing files, taking a photo, taking a video, and so on.
Let's take a look at different ways to set up the file input.
Differences in file input behaviour between iOS and Android
Although the following is not an exhaustive list of ways to set up the file input element, these are a pretty good set of examples that we could default to.
NOTE: The examples below use Angular event bindings to handle the change
event, but otherwise the implementation will be the same with vanilla JavaScript, StencilJS, React, Vue, or whatever else you are using.
Standard File Input
<input type="file" (change)="getFile($event)" />
On iOS, this will prompt the user to choose between Take Photo or Video
, Photo Library
, or Browse
in order to return the desired file.
On Android, this will directly launch the native file selection screen to select any file on the device.
Limiting File Input to Images
<input type="file" accept="image/*" (change)="getFile($event)" />
On iOS, this will prompt the user to choose between Take Photo
, Photo Library
, or Browse
in order to return the desired file. Note that Video
is no longer a choice in the first option and videos (and other files) will also be excluded from being listed if the user chooses to select an existing photo.
On Android, this will launch the same native file selection screen again, but this time it will be filtered to only show images.
Using Camera for File Input
<input type="file" accept="image/*" capture (change)="getFile($event)" />
On iOS, this will directly launch the camera in Photo
mode and allow the user to take a photo. Once the user takes a photo they will be able to choose whether to use that photo or if they want to retake the photo. Once the user chooses Use Photo
the file will be supplied to the application.
On Android, this will directly launch the camera allowing the user to take a photo (not a video). The user can then accept the taken photo or take another.
Limiting File Input to Videos
<input type="file" accept="video/*" (change)="getFile($event)" />
On iOS, this will prompt the user to choose between Take Video
, Photo Library
, or Browse
in order to return the desired file. Note that Photo
is no longer a choice in the first option and photos (and other files) will also be excluded from being listed if the user chooses to select an existing video.
On Android, this will launch the native file selection screen again, but this time it will be filtered to only show videos.
Limiting File Input to Audio
<input type="file" accept="audio/*" (change)="getFile($event)"
On iOS, this will prompt the user to choose between Take Photo or Video
, Photo Library
, or Browse
in order to return the desired file. Note that there is no restriction to audio files only in this case.
On Android, this will launch the native file selection screen again, but this time it will be filtered to only show audio files.
Keep in mind that the specification for the file input element has changed over the years, so you might find many different examples of ways to set up this element and force certain behaviours. In general, my advice would be not to try to "game the system". Use the simplest options and focus on telling the browser what you want, then let the platform decide how best to fulfill that request. If you try to get too tricky and take over this process to enforce what you want, you will leave yourself vulnerable to different behaviours on different platforms/versions and also your solution will be more prone to breaking in the future.
If you do need more control over this process, in ways that using the file input element does not allow (or at least it does not allow it consistently across platforms), you can look into using native plugins/APIs instead. The Camera API, for example, will give you much greater control over the process of selecting/capturing a photo than the <input type="file">
element will.
How do we upload these files to a server?
Fortunately, the resulting file reference can be uploaded in the same way as a file retrieved from a normal desktop/web file input element. You will just need to make a POST
request that includes multipart/form-data
that contains the file(s) you want to upload. For more details on doing that, check out the previous tutorial: Handling File Uploads in Ionic.
Summary
The standard <input type="file">
element provides a surprisingly smooth file selection experience on native iOS and Android platforms, and these files can be easily sent as standard multipart/form-data
to your backend server. This will probably be all you need a lot of the time, but for certain specialised circumstances or use cases you might need to look into using native plugins or APIs to fulfil your file selection and transferring needs.